Introduction
In the world of modern web development, choosing the right stack can make all the difference. Today, we’ll explore a powerful combination: Deno, Prisma, and Turso. This stack offers a TypeScript-first approach, a robust ORM, and a low-latency serverless database, making it perfect for building high-performance apps.
Why Use Deno, Prisma, and Turso? 🤔
- Deno: A secure, modern runtime for JavaScript and TypeScript with built-in support for modules and TypeScript. 🛡️
- Prisma: A next-gen ORM that simplifies database queries and management. Perfect for a TypeScript environment! 📋
- Turso: A serverless SQLite database designed for low-latency, distributed deployments, and real-time data access. 🚀
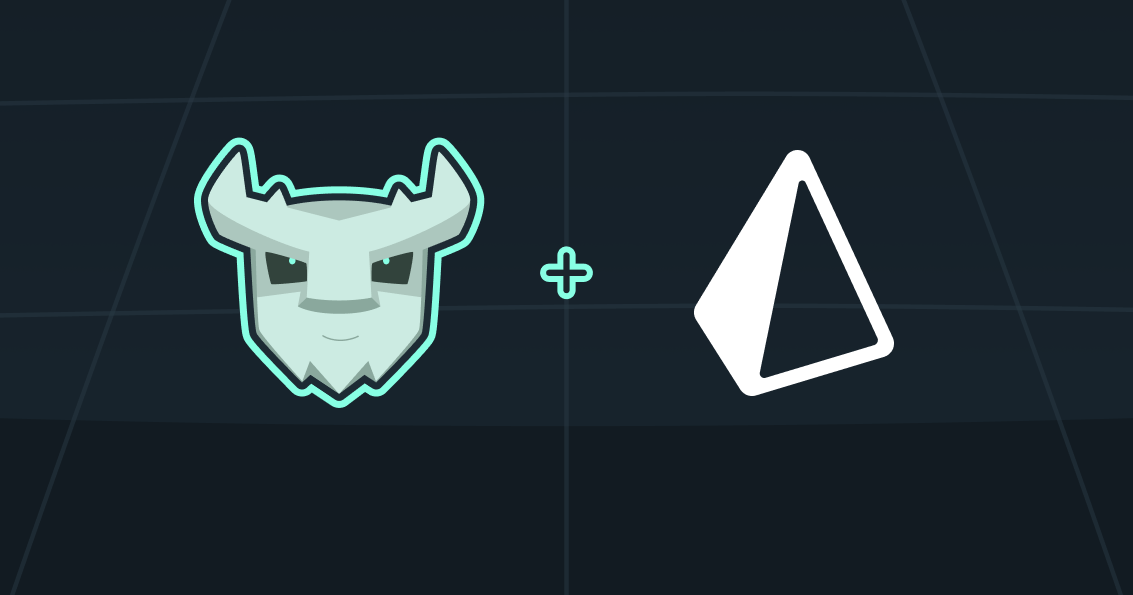
Prerequisites 🛠️
Ensure you have the following installed:
Step 1: Setting Up Deno ⚙️
Initialize a Deno project:
deno init deno-prisma-turso-app
cd deno-prisma-turso-app
Create a main.ts file:
// main.ts
console.log("🚀 Welcome to the Deno, Prisma, and Turso tutorial!");
Run the script:
deno run main.ts
Step 2: Integrating Prisma with Deno 🗂️
While Prisma doesn’t natively support Deno yet, we can still use the Prisma client generated by Node.js.
1. Initialize Prisma:
deno run -A npm:prisma init
2. Define your database schema in prisma/schema.prisma:
datasource db {
provider = "sqlite"
url = "file:../data/demo.db"
}
generator client {
provider = "prisma-client-js"
output = "../generated/client"
previewFeatures = ["driverAdapters"]
}
model User {
id Int @id @default(autoincrement())
name String
email String @unique
}
3. Generate the Prisma Client:
deno run -A npm:prisma generate
Step 3: Setting Up Turso 🗄️
Turso is a serverless SQLite database designed for performance.
1. Create a Turso database:
turso db create my_database
2. Set the database URL in your .env file:
DATABASE_URL="turso://<your-database-url>"
3. Push the schema to your Turso database:
deno run -A npm:prisma db push
Step 4: Writing Deno Code to Interact with Turso via Prisma 💻
Here’s a script to connect Deno with your Turso database using Prisma:
// client.ts
import { createRequire } from "node:module";
import { type PrismaClient } from "../generated/client/index.d.ts";
import { PrismaLibSQL } from "npm:@prisma/adapter-libsql";
import { createClient } from "npm:@libsql/client/node";
const require = createRequire(import.meta.url);
export const Prisma = require("../generated/client/index.js");
export const libsql = createClient({
// url: `file:./data/demo.db`,
syncUrl: Deno.env.get('DATABASE_URL')!,
authToken: Deno.env.get('DATABASE_AUTH_TOKEN')!,
});
const adapter = new PrismaLibSQL(libsql);
export const prisma: PrismaClient = new Prisma.PrismaClient({
adapter,
log: ["query", "info", "warn", "error"],
});
export async function getUsers() {
const users = await prisma.user.findMany();
return users;
}
export async function createUser(name: string, email: string) {
const user = await prisma.user.create({
data: { name, email },
});
return user;
}
Step 5: Testing Your Deno App ✅
Create a test.ts file to verify the database integration:
import { createUser, getUsers } from "./client.ts";
const user = await createUser("John Doe", "[email protected]");
console.log("👤 User created:", user);
const users = await getUsers();
console.log("📋 All users:", users);
Run the test script:
deno run --allow-env --allow-read --allow-net test.ts
Conclusion 🎉
Congratulations! You’ve successfully built a modern, serverless app using Deno, Prisma, and Turso. This stack offers a TypeScript-first approach, powerful ORM capabilities, and a fast, distributed database—all crucial components for building scalable, high-performance applications. 🌐
By using Deno’s secure runtime, Prisma’s intuitive ORM, and Turso’s low-latency database, you’re well-equipped to handle complex data needs while keeping your codebase clean and efficient. 🚀
What’s Next? 🛤️
- Explore Advanced Prisma Features: Dive deeper into complex queries, migrations, and relations.
- Integrate Front-end Frameworks: Consider using Hono, React, or Svelte for building the front end of your app.
- Experiment with Turso: Explore real-time updates and distributed deployments to optimize data access.
Need Help? Contact Us! 📩
If you need assistance or have questions about this stack, don’t hesitate to reach out to Gleez. Our team of experts can help you leverage Deno, Prisma, and Turso for your next project. Let’s build something amazing together! 🚀