🚀 Introduction
When you’re dealing with multi-tenant databases in MySQL, efficiently managing large datasets with non-uniform distribution across tenants is a critical task. In a scenario with 10,000 tenants, some of which have much more data than others, using a strategy like partitioning is crucial to ensure both performance and scalability.
Partitioning can help optimize query performance and manage large tables better by reducing the amount of data the database has to scan. But how do you approach partitioning when your tenants are non-uniform in terms of data load?
In this post, we’ll explore different partitioning strategies for multi-tenant systems and provide best practices for ensuring efficient data management in MySQL.
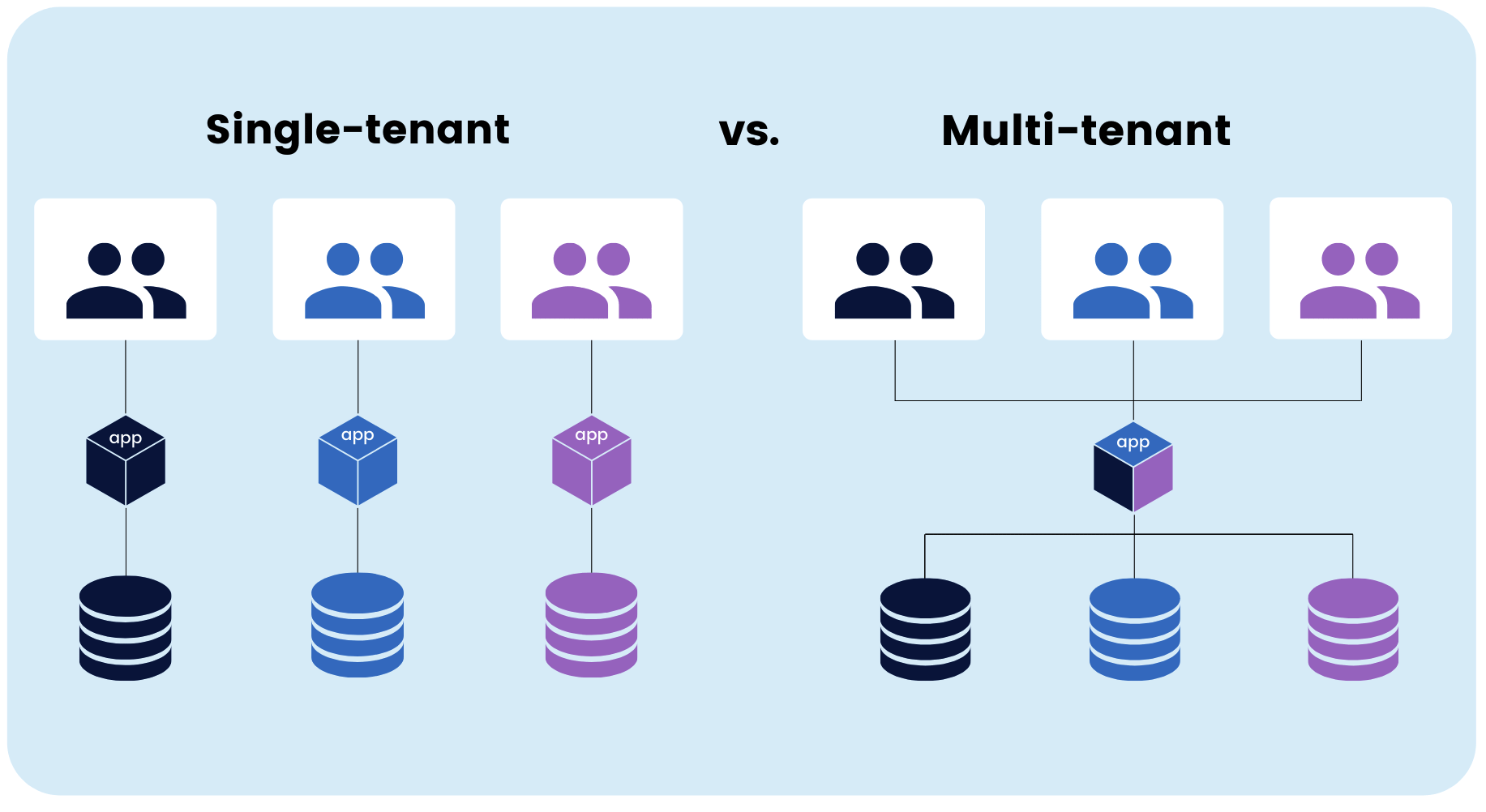
🛠️ Recommended Partitioning Strategies
1. Partition by Tenant Groups 🏢
One of the most practical approaches for handling 10,000 tenants is partitioning by tenant groups. When you have tenants with non-uniform data sizes, you can group them based on size or any logical grouping criteria (e.g., region, subscription type).
How It Works:
- Small tenants with less data go into one partition.
- Medium tenants with moderate data go into another partition.
- Large tenants with significant data are placed in a different partition.
This helps balance the data load across partitions and ensures efficient queries.
CREATE TABLE tenant_data (
tenant_id INT,
data VARCHAR(255),
created_at DATETIME
)
PARTITION BY RANGE (tenant_id) (
PARTITION p_small VALUES LESS THAN (2000), -- Small tenants (ID < 2000)
PARTITION p_medium VALUES LESS THAN (7000), -- Medium tenants (ID < 7000)
PARTITION p_large VALUES LESS THAN MAXVALUE -- Large tenants (ID > 7000)
);
By using range-based partitioning, MySQL can easily scan only the relevant partition during queries, improving performance.
2. Partition by Tenant Type or Region 🌍
Another approach is partitioning based on tenant types (e.g., free, premium) or regions (e.g., North America, Europe). This method is useful when tenant data is related to certain geographic or business characteristics.
How It Works:
- Tenants from the same region or business type are grouped into specific partitions.
CREATE TABLE tenant_data (
tenant_id INT,
region VARCHAR(255),
data VARCHAR(255),
created_at DATETIME
)
PARTITION BY LIST COLUMNS (region) (
PARTITION p_region_north VALUES IN ('North America'),
PARTITION p_region_europe VALUES IN ('Europe'),
PARTITION p_region_asia VALUES IN ('Asia'),
PARTITION p_region_rest VALUES IN ('Other')
);
This can be beneficial when you need to apply localization or region-specific optimizations.
3. Hash Partitioning for Even Data Distribution 🎲
If tenants’ data sizes are extremely varied and uneven, hash partitioning can be a great solution. By hashing the tenant ID, you can distribute tenants randomly across multiple partitions to balance the load.
How It Works:
- Tenant data is spread evenly across partitions by hashing the tenant ID.
CREATE TABLE tenant_data (
tenant_id INT,
data VARCHAR(255),
created_at DATETIME
)
PARTITION BY HASH(tenant_id)
PARTITIONS 20; -- Spread across 20 partitions
This method ensures that no partition becomes a bottleneck, making it ideal for unpredictable or uneven data loads.
4. Hybrid Approach: Range + Hash 🔀
For even better control over partitioning, you can combine both range and hash partitioning. This hybrid method allows you to logically group tenants by size or region, while ensuring balanced data distribution within each partition using hashing.
How It Works:
- Range-based partitioning separates tenants by groups.
- Hashing ensures even distribution within each group.
CREATE TABLE tenant_data (
tenant_id INT,
data VARCHAR(255),
created_at DATETIME
)
PARTITION BY RANGE (tenant_group)
SUBPARTITION BY HASH(tenant_id)
SUBPARTITIONS 10 (
PARTITION p_small VALUES LESS THAN (5000),
PARTITION p_large VALUES LESS THAN MAXVALUE
);
This approach gives you the best of both worlds: logical grouping with even data distribution.
🤔 Which Strategy is Best for You?
- If you have tenants with similar data size, use hash partitioning to evenly distribute data.
- For tenants with varying data sizes, group them by size or region using range partitioning.
- If you need even more control, use the hybrid approach with range and hash partitioning.
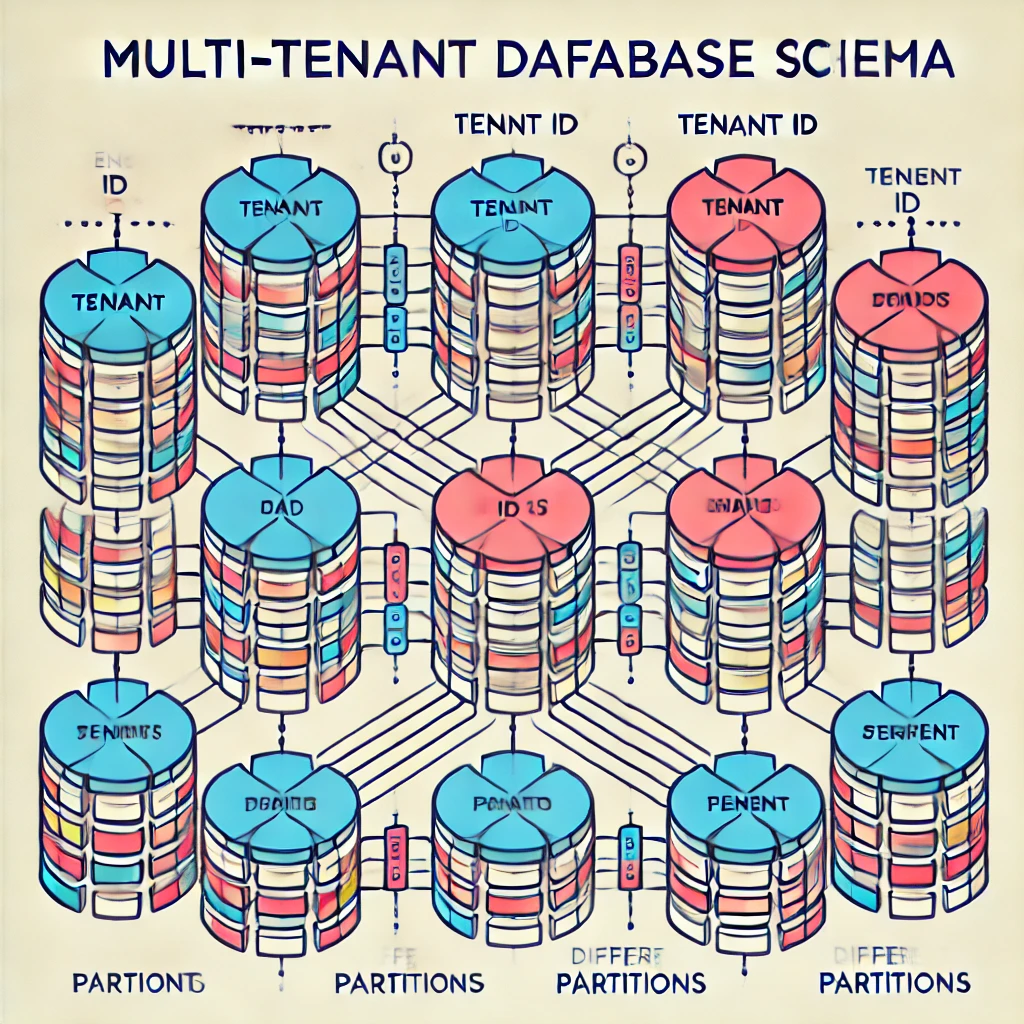
💡 Conclusion
Partitioning is a powerful way to handle large datasets efficiently in MySQL, especially when dealing with multi-tenant architectures. By grouping tenants logically and distributing data evenly, you can improve both performance and manageability.
For a setup with 10,000 tenants and non-uniform data, the best approach is to partition by tenant groups based on size or characteristics, or use hash partitioning for even distribution. Combining both strategies in a hybrid approach can give you optimal performance and flexibility.
Take the time to evaluate your data structure and pick the best strategy for your system!
⚙️ Related Topics:
- MySQL Partitioning Best Practices
- Scaling Multi-Tenant Systems
- Optimizing Database Performance with Partitioning
👨💻 Happy coding and optimizing!